DirecrX (Managed) Create a Device C#
C# 2007. 10. 30. 15:17 |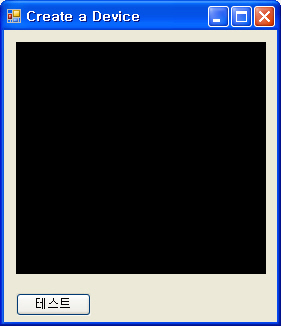
C#에서 프로젝트를 하기 위해서 DirectX를 연동하고 있다.
현재 소스는 Tutorial 1: Create a Device를 사용해서 UserControl에 붙인 것과 동일합니다.
----DxViewer.cs----
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Drawing;
using System.Data;
using System.Linq;
using System.Text;
using System.Windows.Forms;
// DirectX
using Microsoft.DirectX;
using Microsoft.DirectX.Direct3D;
using Direct3D = Microsoft.DirectX.Direct3D;
namespace Gsi.DxViewer
{
public delegate void RenderEventHandler(object sender, DeviceEventArgs e);
public delegate void CreateDeviceEventHandler(object sender, DeviceEventArgs e);
public class DeviceEventArgs : EventArgs
{
private Device _device;
public Device Device { get { return _device; } }
public DeviceEventArgs(Device device)
{
_device = device;
}
}
public partial class DxViewer : UserControl
{
#region Members;
private Device _device = null;
// private Form _parent = null;
public event RenderEventHandler OnRender;
public event CreateDeviceEventHandler OnCreateDevice;
#endregion
#region ctor
public DxViewer()
{
InitializeComponent();
}
#endregion
#region Init DX
/// <summary>
/// Init the DirectX-Stuff here
/// </summary>
/// <param name="parent">parent of the DevicePanel</param>
///
public void Init()
{
try
{
// Setup our D3D stuff
PresentParameters presentParams = new PresentParameters();
presentParams.Windowed = true;
presentParams.SwapEffect = SwapEffect.Discard;
_device = new Device(0, DeviceType.Hardware, this,
CreateFlags.SoftwareVertexProcessing, presentParams);
if (OnCreateDevice != null)
OnCreateDevice(this, new DeviceEventArgs(_device));
}
catch (DirectXException ex)
{
throw new ArgumentException("Error initializing DirectX", ex);
}
}
#endregion
#region Properties
/// <summary>
/// Extend this list of properties if you like
/// </summary>
private Color _deviceBackColor = Color.Black;
public Color DeviceBackColor
{
get { return _deviceBackColor; }
set { _deviceBackColor = value; }
}
#endregion
#region Rendering
/// <summary>
/// Rendering-method
/// </summary>
public void Render()
{
if (_device == null)
return;
//Clear the backbuffer
_device.Clear(ClearFlags.Target, _deviceBackColor, 1.0f, 0);
//Begin the scene
_device.BeginScene();
// Render of scene here
if (OnRender != null)
OnRender(this, new DeviceEventArgs(_device));
//End the scene
_device.EndScene();
_device.Present();
}
#endregion
#region Overrides
protected override void OnPaint(PaintEventArgs e)
{
// Render on each Paint
this.Render();
}
#endregion
}
}
----Form1.cs----
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
// DirectX
using Microsoft.DirectX;
using Microsoft.DirectX.Direct3D;
using Direct3D = Microsoft.DirectX.Direct3D;
using Gsi.DxViewer;
namespace AzeViewerSimple2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
dxViewer1.Init();
}
private void button1_Click(object sender, EventArgs e)
{
dxViewer1.Invalidate();
}
private void dxViewer1_OnCreateDevice(object sender, DeviceEventArgs e)
{
Device device = e.Device;
}
private void dxViewer1_OnRender(object sender, DeviceEventArgs e)
{
Device device = e.Device;
}
}
}
----참고사항----
References 에
Microsoft.DirectX
Microsoft.DirectX.Direct3D
Microsoft.DirectX.Direct3DX
를 추가 한후에 빌더를 하고 나면 DxViewer 컨트롤이 Toolbox에 생깁니다.
그 컨트롤을 Form1의 디자인 화면으로 드래그 해서 넣은 후에
이름을 dxViewer1이라고 지정한후 코드를 참조 하시면 됩니다.
이후 질문은 코멘트 달아 주세요.