[C#] ListBox DrawItem 에 대한 의견..
C# 2008. 8. 23. 01:22 |맨날 도구상자에 있는 컨트롤만 쓰고 있는 나로서는..
새로운 UI를 구상할때면.. 좀 난감해 지네요 ^^..
이번에는 ListBox를 확장해야할듯 해서
바로 위와 같이 하나의 아이템에 복합적인 데이터가 들어가있다고 가정을 하고 작업을 해야 됩니다.
이미지 정보와 해당 이름 또는 나이 저노하 번호 등등..
이걸 위해서는 DrawItem()을 따로 구현해 줘야 되네요.
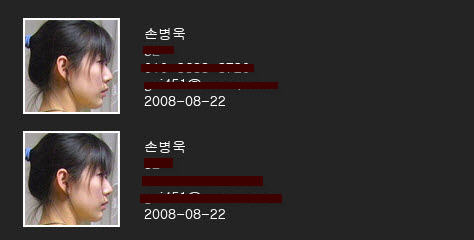
우선 데이터를 집어 넣을때는
listBox1.Items.Add(new TestTemplate("손병욱", "d:\\a.bmp"));
이와 같이 하나의 데이터 템플릿 클래스를 제작하고 그기에 맞도록 해당 데이터를 추가 해야 됩니다.
그리고 DrawItem()을 가능하게 하기 위한 ListBox의 설정또한 잊지 말아야 하구요.. ^^
간단하게 DrawItem의 드로잉 하는 부분을 아래와 같이 처리 하면 되는군요.
{
if(listBox1.Items.Count <= 0)
return;
// Set the DrawMode property to draw fixed sized items.
listBox1.DrawMode = DrawMode.OwnerDrawVariable;
// Draw the background of the ListBox control for each item.
e.DrawBackground();
// Create a new Brush and initialize to a Black colored brush by default.
Brush myBrush = Brushes.Black;
// Determine the color of the brush to draw each item based on the index of the item to draw.
switch (e.Index)
{
case 0:
myBrush = Brushes.Red;
break;
case 1:
myBrush = Brushes.Orange;
break;
case 2:
myBrush = Brushes.Purple;
break;
}
// 이 부분에서 해당 값을 가져 올때 TestTemplate의 값으로 형변환 한 후에 값을 접근가능..
TestTemplate tt = (TestTemplate)listBox1.Items[e.Index];
// Draw the current item text based on the current Font and the custom brush settings.
e.Graphics.DrawImage(new Bitmap(tt.path), e.Bounds);
e.Graphics.DrawString(tt.name, e.Font, myBrush,e.Bounds,StringFormat.GenericDefault);
// If the ListBox has focus, draw a focus rectangle around the selected item.
e.DrawFocusRectangle();
}
이때 이미지와 여러가지를 표현하기 위해서는 아래의 함수도 오버라이딩 해서 변경해줘야 하네요.
{
e.ItemHeight = 100;
}
위의 내용을 토대로 샘플을 하나 만들어 볼까 합니다.
하지만 고려되어야 하는 사항은 이미지가 들어가기 때문에
많은 양의 데이터를 읽어 들일때 UI가 블락킹 되는 현상이 불현듯 보이네요..
백그라운드 쓰레드나 일반 쓰레드를 통해서 따로 돌려야 할듯 합니다.
그러면 조금더 나은 UI 및 컨트롤을 구현할 수 있을거 같습니다.
개봉박두~~~..